Tutorial API Pytest
Hello guys, we will learn about python framework pytest for automation test api. Pytest is one of popular framework pyhton test, besides the robot framework. We will learn learn the robot framework in next article, after finish some article pytest. Why pytest? Because The pytest framework makes it easy to write small, readable tests, and can scale to support complex functional testing for applications and libraries. In pytest we will use virtual environment for run test.
In this tutorial, we will use api https://jsonplaceholder.typicode.com/posts
Before start, please make sure you have been download, install python, and install pip. If there are no python, visit https://www.python.org/downloads/.
1. Open text editor (eg visual studio code, sublime, atom, etc)
2. Click File, Click Open Folder
3. Choose directory you wanna save your folder, click create new folder, rename folder, click open folder (example api-pytest folder).
4. Open terminal in text editor (ctrl + ‘)
5. Create virtual environment
python -m venv venv
6. Activate virtual environment
.\venv\Scripts\activate
7. Install dependencies
pip install pytest requests
8. Create folder tests and create file test_api.py
We will test 5 scenarios : get all, get by id, create, update, and delete.
9. Define import & url in top line of code
import pytest
import requests
from jsonschema import validate, ValidationError
url = 'https://jsonplaceholder.typicode.com/posts'
10. Create test get all in test_api.py
def test_get_all():
response = requests.get(url)
body = response.json()
# Memvalidasi response status code 200
assert response.status_code == 200
# Memvalidasi setiap item dalam array hasil respons dengan skema
for item in body:
try:
validate(instance=item, schema=expected_schema)
except ValidationError as e:
pytest.fail(f"JSON tidak valid: {e.message}")
in test get all we validate response status code & response json scema. You able to convert response json to json schema in https://jsonformatter.org/json-to-jsonschema.
11. Create test get by id in test_api.py
def test_get_by_id1():
# response = requests.get(url)
response = requests.get(f"{url}/1")
body = response.json()
# Memvalidasi response status code 200
assert response.status_code == 200
# Memvalidasi bahwa id = 1 ada dalam respons
assert body["id"] == 1
# Memvalidasi properti lainnya ada dalam respons
assert "userId" in body
assert "title" in body
assert "body" in body
# Memvalidasi nilai yang diharapkan
assert isinstance(body["userId"], int)
assert isinstance(body["id"], int)
assert isinstance(body["title"], str)
assert isinstance(body["body"], str)
in test get by id, there are a bit different of url because what id will be show. We validate response status code, value, property in response body.
12. Create test_post in test_api.py
def test_post():
post_data = {
"title": "pytest",
"body": "learning pytest is really fun",
"userId": 1
}
# Mengirim permintaan POST ke API
response = requests.post(url, json=post_data)
# Memvalidasi status code adalah 201 (Created)
assert response.status_code == 201
# Mendapatkan body JSON dari respons
body = response.json()
# Memvalidasi bahwa respons memiliki data yang dikirim
assert body["title"] == post_data["title"]
assert body["body"] == post_data["body"]
assert body["userId"] == post_data["userId"]
# Memvalidasi bahwa ID baru telah dibuat (otomatis oleh API)
assert "id" in body
assert isinstance(body["id"], int)
# Memvalidasi nilai ID lebih besar dari 0 (valid ID)
assert body["id"] > 0
in test post, there are a bit different, because post we must include data in json. we validate response status, value & property in respose body.
13. Create test_put in test_api.py
def test_update_id1():
put_data = {
"title": "python & pytest",
"body": "learning python & pytest is really fun",
"userId": 1
}
# Mengirim permintaan PUT ke API
response = requests.put(f"{url}/1", json=put_data)
# Memvalidasi status code adalah 200 (Ok)
assert response.status_code == 200
# Mendapatkan body JSON dari respons
body = response.json()
# Memvalidasi bahwa respons memiliki data yang dikirim
assert body["title"] == put_data["title"]
assert body["body"] == put_data["body"]
assert body["userId"] == put_data["userId"]
# Memvalidasi bahwa ID baru telah dibuat (otomatis oleh API)
assert "id" in body
assert isinstance(body["id"], int)
# Memvalidasi nilai ID lebih besar dari 0 (valid ID)
assert body["id"] > 0
in test post, there are a bit different with url, because we must what id will be updated. we validate response status, value & property in respose body.
14. Create test_delete in test_api.py
def test_delete_id1():
# Mengirim permintaan delete ke API
response = requests.delete(f"{url}/1")
# Memvalidasi status code adalah 200 (Ok)
assert response.status_code == 200
in test delete, there are a bit different with url, because we must specific what id will be deleted. we validate response status.
15. Create file pytest.ini in folder api-pytest
[pytest]
markers =
api
testpaths = tests
it is for marker test & filter run test
16. Run test
python -m pytest -k "api"
We will see all test passed like this
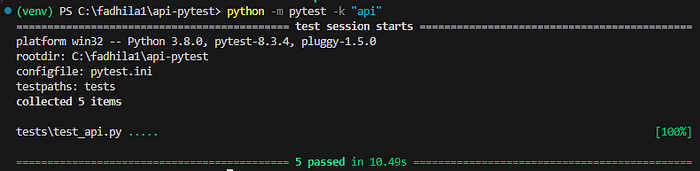
That’s all from this tutorial, wish help you to learn automation. Visit repo this project https://github.com/fadhilara/api-pytest. If any error & feedback, please drop in comment.